ls -l
is a relatively simple and straightforward command. But what actually
takes place behind the screen will get you to appreciate how little work
is required from you to acquire the output you desire.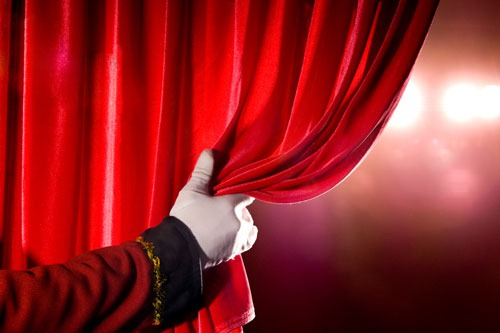
ls
is a shell command that lists files and directories within a directory. With the -l
option, ls
will list out files and directories in long list format. You will encounter something like this: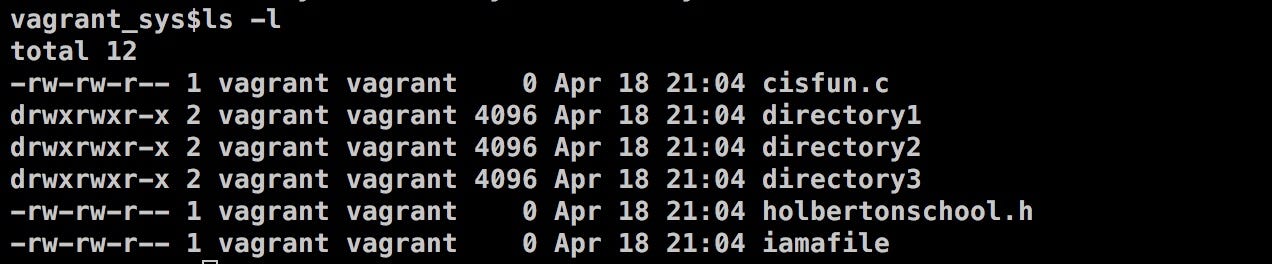
To understand how shell interpret a user’s input, it helps to get a basic understanding of how an operating system is organized.
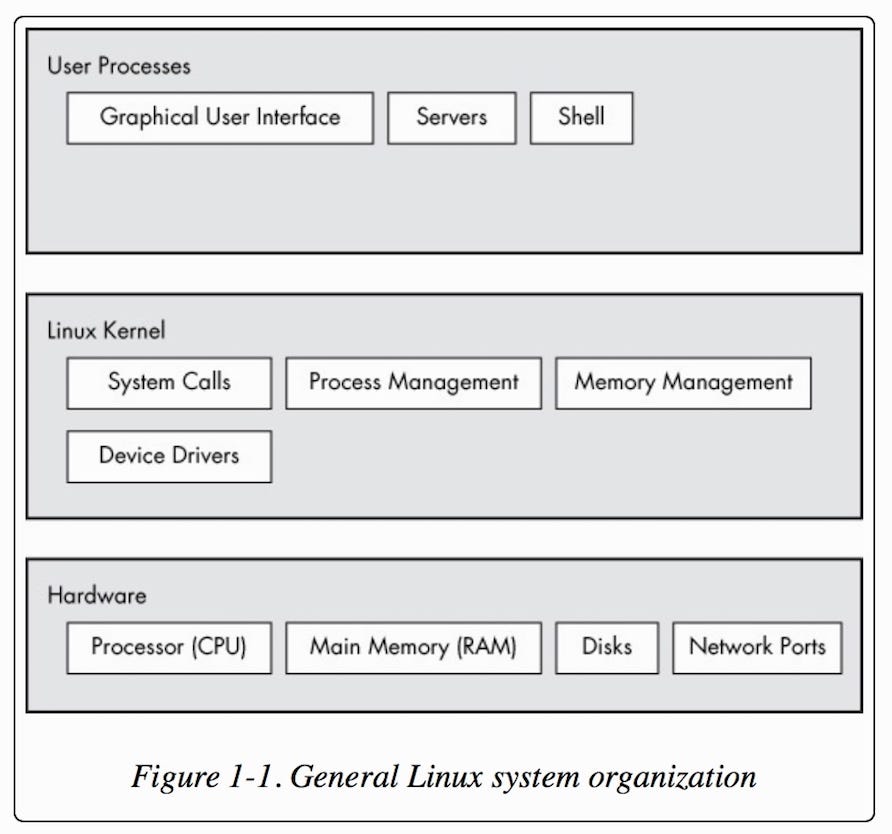
An operating system can be broken down into 3 main chunks: Hardware holds the main memory, controls the CPU, disks, network interfaces, etc. Following hardware is kernel, which is the core of the operating system. It acts as an interface between hardware and any running program. User interface sits in the user process level. This is what you see on your screen.
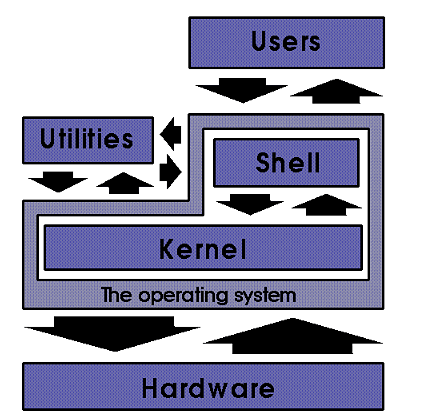
The kernel
is also responsible for determining which processes are allowed to use
the CPU as well as memory and system calls management. We can’t and
shouldn’t be trusted to access kernel directly, because we can screw
things up royally on accident.
But programs in the user’s space can interact with the kernel via the kernel feature, system calls(syscalls).
System calls allow applications to communicate with the kernel. An
application can call functions in a library that rely on the system call
interface to instruct the kernel to execute on behalf of the
application.
Computers
understand binary language made up of 0’s and 1’s. Instead of figuring
out the binary code for a command(good luck), user’s input is translated
to machine readable language via a command line interpreter. Shell is a
type of command line interpreter — an interface to the kernel that
allows user to execute commands in the operating system.
What happens when you type ls -l
and hit enter — in plain English:
Everything is a file in Linux and other UNIX-like operating systems. The command
ls
is a file containing the program to execute the ls
command. So when you type in a command, shell will attempt to locate
the command(file) by searching through its disk and directories in your
PATH. If the program is found, shell will clone itself and run the
corresponding program in the clone/new process. The cloned process will
then be replaced with the program it wants to run, and the original
process will wait until the new process completes its execution before
moving forward. The output can be written in standard output, which is
what you see in your terminal. It can also be piped, or redirected, into
a file or even to another command. When we type ls
and hit enter, we are typing our command from the standard input.
Similarly, you can invoke the command by having it piped from a file or a
command. After the command is executed, control will be returned to the
original process and you will be greeted with the login shell, once
again.
What happens when you type ls -l
and hit enter — in C
Steps to processing a user command:
- Get user input
- Check for expansions and alias.
- Check builtin.
- Check PATH.
- If file exists: fork and execute program in the child process.
- After child process terminates, parent process will print prompt to user.
Get user input:
Upon entering shell, you will encounter the prompt(prompt string 1, or PS1). After you input
ls -l
to the command line, shell reads your input by using getline()
(man 3 getline). Getline reads from the standard input file stream, STDIN, and stores the user input into a buffer as a string.
The buffer(containing the input
"ls -l"
) is then broken down into tokens and stored in an array: {"ls", "-l", "NULL"}
. args[0] = "ls", args[1] = "-l", args[2] = "NULL"
Check for expansions and alias:
Prior to searching for the
ls
command, shell will check for special characters that need to be expanded(i.e., *, $, etc). For example, if the user input is ls *.c
, this is when *.c
will be replaced with all .c
files within current directory. Shell also checks for aliases(an alias in shell is similar to a keyboard shortcut). If ls
is an alias, shell will replace ls
with its corresponding value here. Say we set up an alias called apple: alias apple="cat"
. After entering apple main.c
, our command will be processed as cat main.c
.
Check builtin:
If
ls
is not an alias, shell will then check whether the command ls
is a built-in command. A built-in command is a command that is built into and executed in shell itself. Examples: cd
, echo
, alias
, help
, read
, type
. Additional bash builtins.
Check PATH:
If
ls
is not a builtin, shell will look for ls
in the environment variable, PATH. First, a copy of PATH will be tokenized(separated by :
)
with each representing the path to a directory. A copy is necessary
here because we don’t actually want to alter PATH. We want to butcher a
copy of PATH into tokens in order to check against the entered command.
Now shell will append/ls
— slash + our tokenized input — to the end of each directory listed in PATH to check whether or not the file exists.
The tokens are delimited by a colon, so here, the user input will be checked against
/usr/local/sbin
, /usr/local/bin
, usr/sbin
, usr/bin
, etc./ls
will be appended to the end of each token: /usr/local/sbin/ls
, /usr/local/bin/ls
, etc.
After searching through each token, an error will occur if
ls
is not an existing file.
Fork and execute program in the child process:
Upon locating the
ls
file in PATH, shell will open and run the file ls
by calling fork()
. fork()
is a system call for creating a clone of the current process. All user processes on a Linux system stem off as a result of fork()
. Both processes will run the instructions following fork()
. So to distinguish from the two processes,fork()
returns the child’s process id to the parent, 0 in the child process, and -1 to indicate an error.- Shell(calling process/parent process)will call
fork()
to create a copy of itself(child process). The clone/child process will have its own system process ID. Running a program in a separate process protects the parent/current process should the program cause any problems upon execution. - The child process then calls
execve()
to run the user’s command,ls
.execve()
will replace the current(child) process with the program it calls(ls
, in this case). - Parent process waits until the child competes its execution via
wait()
.
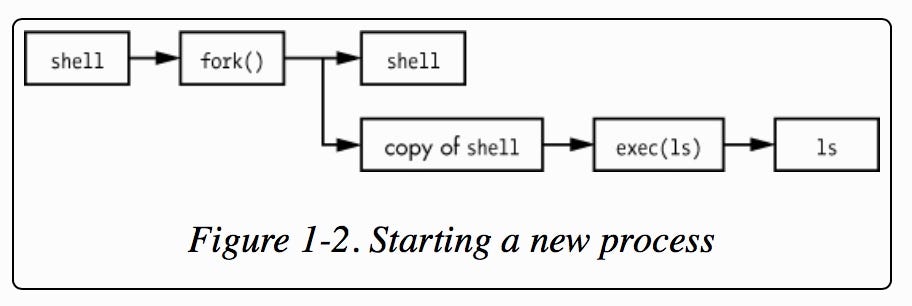
Upon
completion, the child process will terminate and control will be
returned back to the parent process. You will be greeted with the prompt
once
ls
is executed and will be able to enter the next command :)
When
you log on to your system, shell login maintains an environment in
which you can run programs. An environment contains environment
variables that provide information about the system behavior. Inputting
env
on the command line will print a list of environment variables to standard output. Inputting echo $PATH
will print out the specified environment variable — in this case, PATH.
When
you type in a command, shell will search in a list of directories to
see whether that program/file exists. This list of directories is stored
in the environment variable PATH, and it’s set when you log on. More
on — Environment Variable, PATH.
Comments
Post a Comment
https://gengwg.blogspot.com/